During the past year and as part of our philosophy of continuous improvement, we have been conducting various shared-training sessions, in which our team members themselves share their knowledge, experiences and ideas. As we know, constant training and continuous learning are essential to keep up to date in a constantly evolving technological world.
In this series of posts, we will discuss some key aspects of these sessions.
Training Pill 02:
Custom Hooks in React
Teach:
Manuel Garre
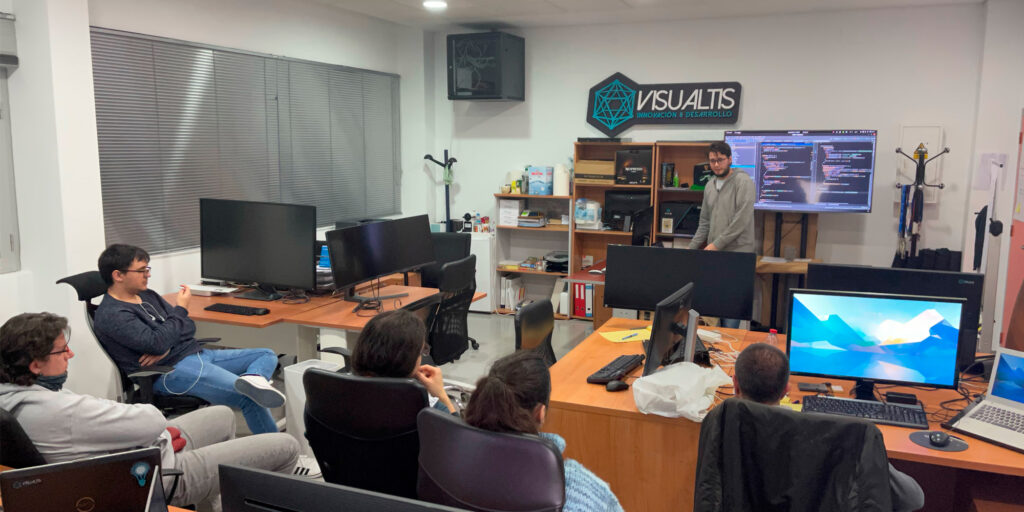
What is a Hook
A Hook is a javascript function that allows to create/access the state and life cycles of React and that, to ensure the stability of the application, must be used following two basic rules:
– It must be called at the top level of the application, it must never be called inside cycles, conditionals or nested functions, since the order in which hooks are called must always be the same to ensure that the result is predictable during rendering. This top-level-only usage is what ensures that React’s internal state is correctly preserved between different calls of the same hook.
– It should be called in functions or other custom React hooks, so that the component’s state logic is clearly visible from the rest of the code for the scope set by React.
Hook examples:
– useState
const [login, setLogin] = useState(”)
const [password, setPassword] = useState(”)
const [hasError, setHasError] = useState(false)
const [view, setView] = useState(View.default)
– useEffect
useEffect(() => {
LoginCas && setView(View.userNotFound)
queryString.includes(‘circleID’) && setView(View.registerUser)
}, [])
How to build custom Hooks?
Building your own Hooks allows you to extract component logic into reusable functions. A custom Hook is a JavaScript function whose name starts with “use” and can call other Hooks.
Example of Custom Hook:
index.jsx
import { useState, useEffect } from “react”;
import ReactDOM from “react-dom”;
const Home = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetch(“https://jsonplaceholder.typicode.com/todos”)
.then((res) => res.json())
.then((data) => setData(data));
}, []);
return (
<>
{data &&
data.map((item) => {
return <p key={item.id}>{item.title}</p>;
})}
</>
);
};
ReactDOM.render(<Home />, document.getElementById(“root”));
We transform to Hook:
useFetch.jsx
import { useState, useEffect } from “react”;
export const useFetch = (url) => {
const [data, setData] = useState(null);
useEffect(() => {
fetch(url)
.then((res) => res.json())
.then((data) => setData(data));
}, [url]);
return [data];
};
index.jsx
import useFetch from “./useFetch”;
const Home = () => {
const [data] = useFetch(“https://jsonplaceholder.typicode.com/todos”);
return (
<>
{data &&
data.map((item) => {
return <p key={item.id}>{item.title}</p>;
})}
</>
);
};